WEBサイト制作・アプリ開発・システム開発・ブランディングデザイン制作に関するご相談はお気軽にご連絡ください。
構想段階からじっくりとヒアリングし、お客様の課題にあわせたアプローチ手法でお客様の“欲しかった”をカタチにしてご提案いたします。
Blog スタッフブログ
iOS
システム開発
[Swift]iOS17から使えるTipKitをUIKitから使ってみた
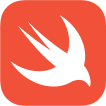
こんにちは、株式会社MIXシステム開発担当のBloomです。
iOS17から利用できる新たなUIパーツとしてTipKitが追加されました。TipKitを使えば簡単にユーザへ新機能の紹介や表示回数などの制御ができるようになります。早速コードで実装してみましょう。
実際に表示してみた
まずはアプリ起動時にTipの表示や表示状態の保存先などの設定を行なってしまいます。AppDelegate.swiftなどでconfigureをコールしてください。
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
try? Tips.configure([
.displayFrequency(.immediate),
.datastoreLocation(.applicationDefault)
])
return true
}
displayFrequencyには表示の頻度を指定し、datastoreLocationには表示フラグの保存先を指定します。
では、実際に表示するTipの設定をしましょう。Tip構造体を継承して表示するTipの宣言をします。
@available(iOS 17.0, *)
struct TipItem: Tip {
var title: Text {
Text("タイトルです")
}
var message: Text? {
Text("ここにメッセージが入ります")
}
var image: Image? {
Image(systemName: "star")
}
}
class ViewController: UIViewController {
@IBOutlet weak var anyButton: UIButton!
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
// この関数を実行することでテスト時に常にTipsを表示させることができます
Tips.showAllTipsForTesting()
let tip = TipItem()
// ここで表示処理を行っています
Task { @MainActor in
for await shouldDisplay in tip.shouldDisplayUpdates {
if shouldDisplay {
let controller = TipUIPopoverViewController(tip, sourceItem: anyButton)
self.present(controller, animated: true)
} else if presentedViewController is TipUIPopoverViewController {
self.dismiss(animated: true)
}
}
}
}
}
実行結果
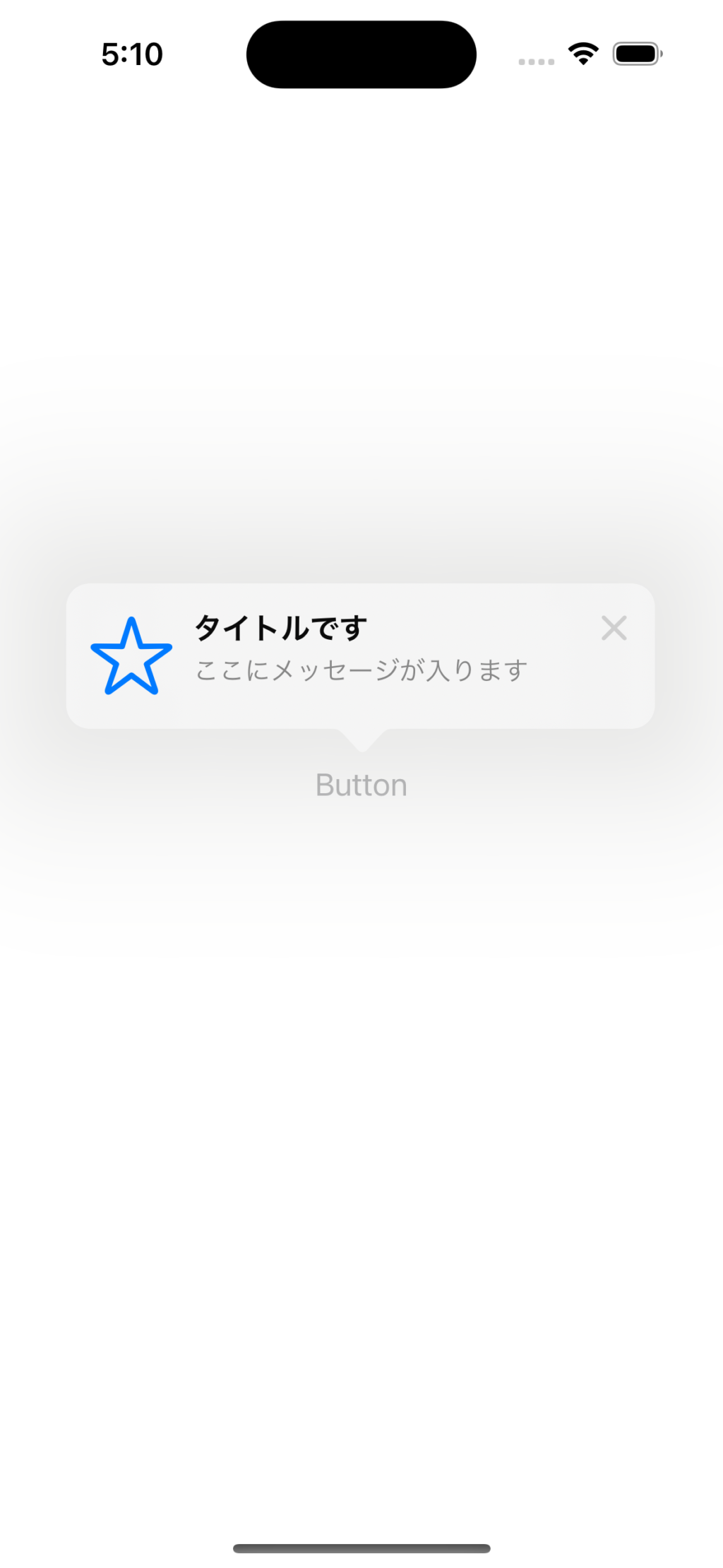
これだけで簡単によくあるTipsの表示をすることができました。このままこのTipにアクションを追加してみましょう。
@available(iOS 17.0, *)
struct TipItem: Tip {
var title: Text {
Text("タイトルです")
}
var message: Text? {
Text("ここにメッセージが入ります")
}
var image: Image? {
Image(systemName: "star")
}
var actionId: String = "actionId"
var actions: [Action] {
[Action(id: actionId, title: "アクション")]
}
}
class ViewController: UIViewController {
@IBOutlet weak var anyButton: UIButton!
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
Tips.showAllTipsForTesting()
let tip = TipItem()
Task { @MainActor in
for await shouldDisplay in tip.shouldDisplayUpdates {
if shouldDisplay {
let controller = TipUIPopoverViewController(tip, sourceItem: anyButton, actionHandler: { action in
guard action.id == tip.actionId else { return }
print("action!")
})
self.present(controller, animated: true)
} else if presentedViewController is TipUIPopoverViewController {
self.dismiss(animated: true)
}
}
}
}
}
実行結果
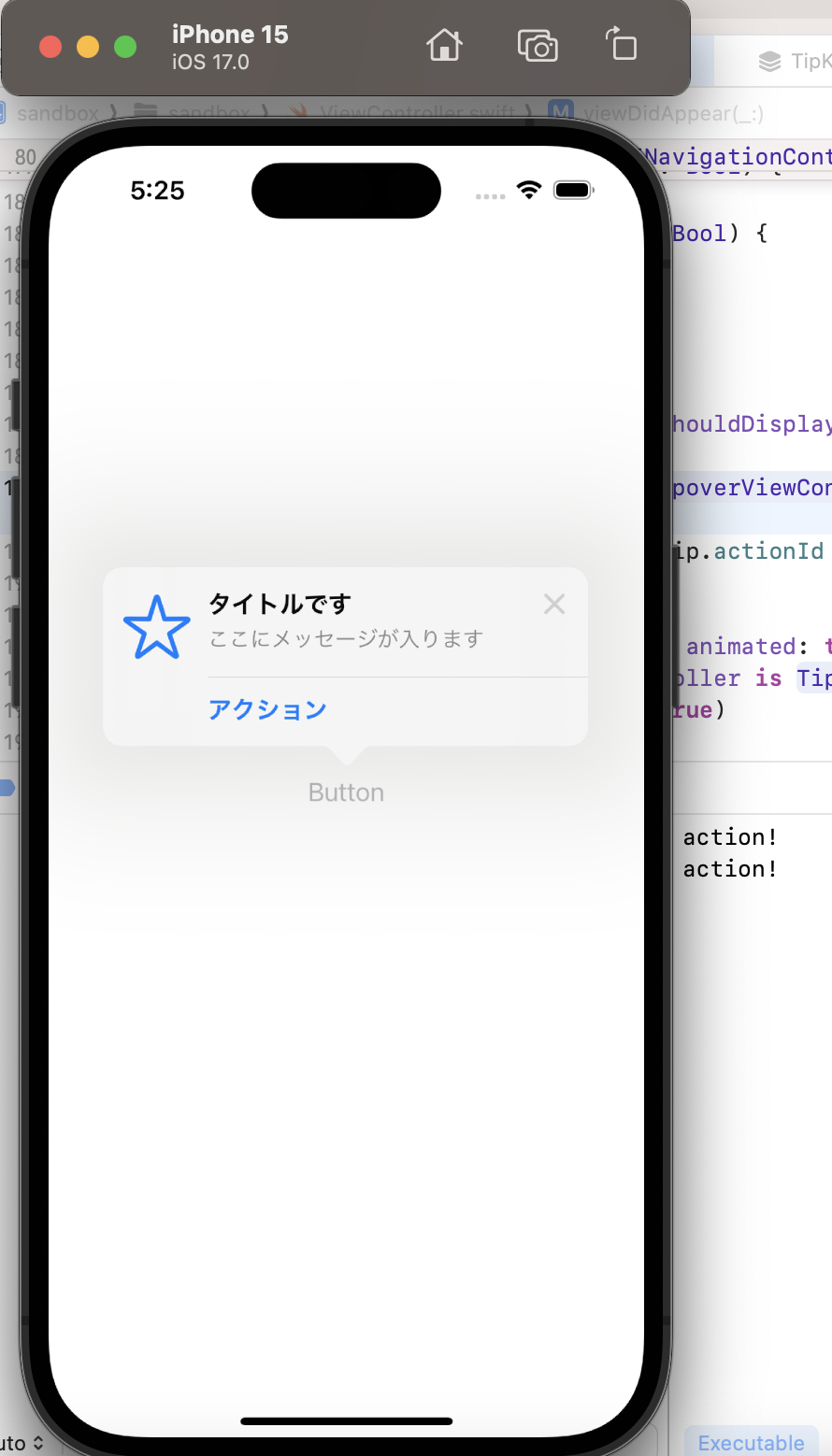
このアクションボタンは押されるだけではTipsが閉じないことを留意しておきましょう。
たったこれだけで簡単にTipsを表示することができました。良かったですね。