Blog スタッフブログ
[Swift]Swagger-CodegenでAPI仕様書からコードを自動生成してみた
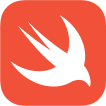
新年あけましておめでとうございます。株式会社MIXシステム開発担当のBloomです。
今回はSwagger-Codegenを利用してOpenAPIに基づきYAMLで記述されたAPI仕様書からSwiftのコードを自動生成してみます。
Swagger-Codegenの導入
Installationの項から環境に適したインストール方法を選びましょう。今回はHomebrewを利用し下記コマンドでインストールを行なった形で進めていきます。
brew install swagger-codegen
コード生成
では、実際にコードを生成してみましょう。こちらはサンプル用に用意したYAMLとなります。
openapi: 3.0.0
info:
title: Sample API
version: 0.1.0
servers:
- url: http://localhost:8000/api/
paths:
/sample:
post:
summary: サンプル
requestBody:
content:
"multipart/form-data":
schema:
properties:
text:
type: string
example: "text"
number:
type: integer
example: 1
required: true
responses:
"200":
description: |
Succeed
content:
"application/json":
schema:
properties:
text:
type: string
example: "戻り値テキスト"
number:
type: integer
example: 2
/lists:
get:
summary: サンプル一覧
responses:
"200":
description: |
Succeed
content:
"application/json":
schema:
properties:
samples:
type: array
items:
allOf:
- $ref: "#/components/schemas/SampleModel"
- type: object
properties:
sample_count:
type: integer
example: 80
"500":
$ref: "#/components/responses/UnexpectedException"
/sample/upload:
post:
summary: アップロード
description: |
サンプル
requestBody:
content:
"multipart/form-data":
schema:
properties:
samples:
type: array
items:
$ref: "#/components/schemas/SampleModel"
required: true
responses:
"200":
description: |
Succeed
"500":
$ref: "#/components/responses/UnexpectedException"
components:
schemas:
SampleModel:
type: object
properties:
id:
type: integer
format: int64
example: 1
name:
type: string
example: "サンプル名"
created:
type: string
format: date-time
example: "2023-01-10 12:00:00"
responses:
UnexpectedException:
description: Unexpected exception
こちらのファイルを保存して下記コマンドを実行します。出力先はoutputディレクトリの指定になります。
swagger-codegen generate -i sample.yaml --lang swift5 -o output
このコマンドを実行することでoutputディレクトリにコードが生成されます。生成されたコードの中から、APIを実行したいSwaggerClientディレクトリを丸ごと投入しましょう。
コードの他にCartfileも生成されていますが、中身はAlamofire 4.9.0を指定する内容なので別途SwiftPMやCarthageで導入しておきましょう。(CarthageでAlamofire5からダウングレードを行う場合、先にCheckoutsからAlamofireを削除しておかないとビルドエラーが発生するようです。)
上記サンプルで生成するとDefaultAPIクラスからAPIをコール可能となります。コード例を記載します。
DefaultAPI.samplePost(text: "text", number: 1) { data, error in
if let data = data {
print(data.text)
print(data.number)
}
else {
print(error)
}
}
DefaultAPI.listsGet { data, error in
if let data = data {
print(data.samples)
}
else {
print(error)
}
}
DefaultAPI.sampleUploadPost(samples: []) { data, error in
if data != nil {
print("succeed")
}
else {
print(error)
}
}
DefaultAPIクラスはSwaggerClient\Classes\Swaggers\APIs\DefaultAPI.swiftで、レスポンスのモデルはSwaggerClient\Classes\Swaggers\Modelsで定義されています。クラス名は自動生成される部分もあるため場合によって適宜書き換えて利用しましょう。
API自体のエントリポイントはSwaggerClient\Classes\Swaggers\APIs.swiftで定義されています。こちらも必要に応じて書き換えを行いましょう。
これでAPI仕様書から直接コードを生成することができました。良かったですね。