WEBサイト制作・アプリ開発・システム開発・ブランディングデザイン制作に関するご相談はお気軽にご連絡ください。
構想段階からじっくりとヒアリングし、お客様の課題にあわせたアプローチ手法でお客様の“欲しかった”をカタチにしてご提案いたします。
Blog スタッフブログ
JavaScript
WEB制作
[jQuery]シンプルなローディング画面の実装
WEB制作担当の木戸です。
ページの読み込み中ローディング画面を表示して、読み込みが完了したら非表示にする方法をご紹介します。
htmlとCSSでローディング画面の見た目を、jQueryで非表示にする処理を作っていきます。
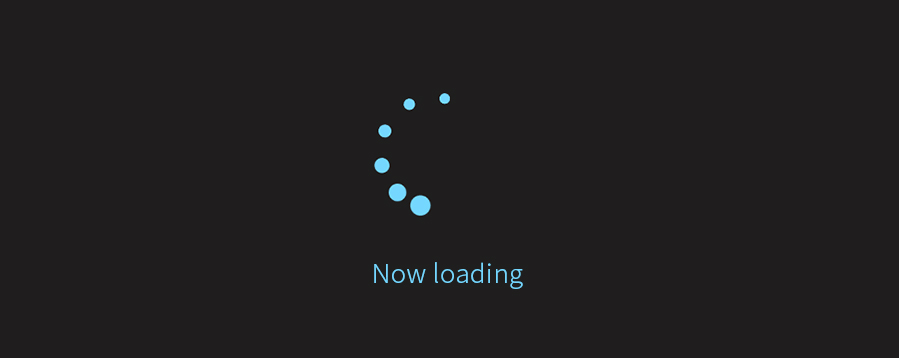
htmlを記述
htmlは上から順番に読み込まれるためなるべく上の方に記述します。
<div id="loading">
<div class="loadingInner">
<!--ここにローディングの中身を記述-->
<div class="sk-chase">
<div class="sk-chase-dot"></div>
<div class="sk-chase-dot"></div>
<div class="sk-chase-dot"></div>
<div class="sk-chase-dot"></div>
<div class="sk-chase-dot"></div>
<div class="sk-chase-dot"></div>
</div>
<p>Now loading</p>
</div>
</div>
CSSを記述
/*ローディング画面背景*/
#loading {
width: 100vw;
height: 100vh;
transition: all 0.3s;
background: #fff;
position: fixed;
top: 0;
left: 0;
z-index: 9999;
}
/*読み込み完了時に非表示*/
.loaded {
opacity: 0;
visibility: hidden;
}
/*画面中央に配置*/
.loadingInner{
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%,-50%);
-webkit-transform: translate(-50%,-50%);
-ms-transform: translate(-50%,-50%);
-moz-transform: translate(-50%,-50%);
}
/*アニメーション*/
.sk-chase {
width: 40px;
height: 40px;
position: relative;
animation: sk-chase 2.5s infinite linear both;
}
.sk-chase-dot {
width: 100%;
height: 100%;
position: absolute;
left: 0;
top: 0;
animation: sk-chase-dot 2.0s infinite ease-in-out both;
}
.sk-chase-dot:before {
content: '';
display: block;
width: 25%;
height: 25%;
background-color: #fff;
border-radius: 100%;
animation: sk-chase-dot-before 2.0s infinite ease-in-out both;
}
.sk-chase-dot:nth-child(1) { animation-delay: -1.1s; }
.sk-chase-dot:nth-child(2) { animation-delay: -1.0s; }
.sk-chase-dot:nth-child(3) { animation-delay: -0.9s; }
.sk-chase-dot:nth-child(4) { animation-delay: -0.8s; }
.sk-chase-dot:nth-child(5) { animation-delay: -0.7s; }
.sk-chase-dot:nth-child(6) { animation-delay: -0.6s; }
.sk-chase-dot:nth-child(1):before { animation-delay: -1.1s; }
.sk-chase-dot:nth-child(2):before { animation-delay: -1.0s; }
.sk-chase-dot:nth-child(3):before { animation-delay: -0.9s; }
.sk-chase-dot:nth-child(4):before { animation-delay: -0.8s; }
.sk-chase-dot:nth-child(5):before { animation-delay: -0.7s; }
.sk-chase-dot:nth-child(6):before { animation-delay: -0.6s; }
@keyframes sk-chase {
100% { transform: rotate(360deg); }
}
@keyframes sk-chase-dot {
80%, 100% { transform: rotate(360deg); }
}
@keyframes sk-chase-dot-before {
50% {
transform: scale(0.4);
} 100%, 0% {
transform: scale(1.0);
}
}
jQueryを記述
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script>
window.onload = function() {
const spinner = document.getElementById('loading');
spinner.classList.add('loaded');
}
</script>
id=”loading”に対して、読み込みが終わったタイミングでclass=”loaded”が追加されます。
消えるときのアニメーションはcssの.loadedで指定しています。